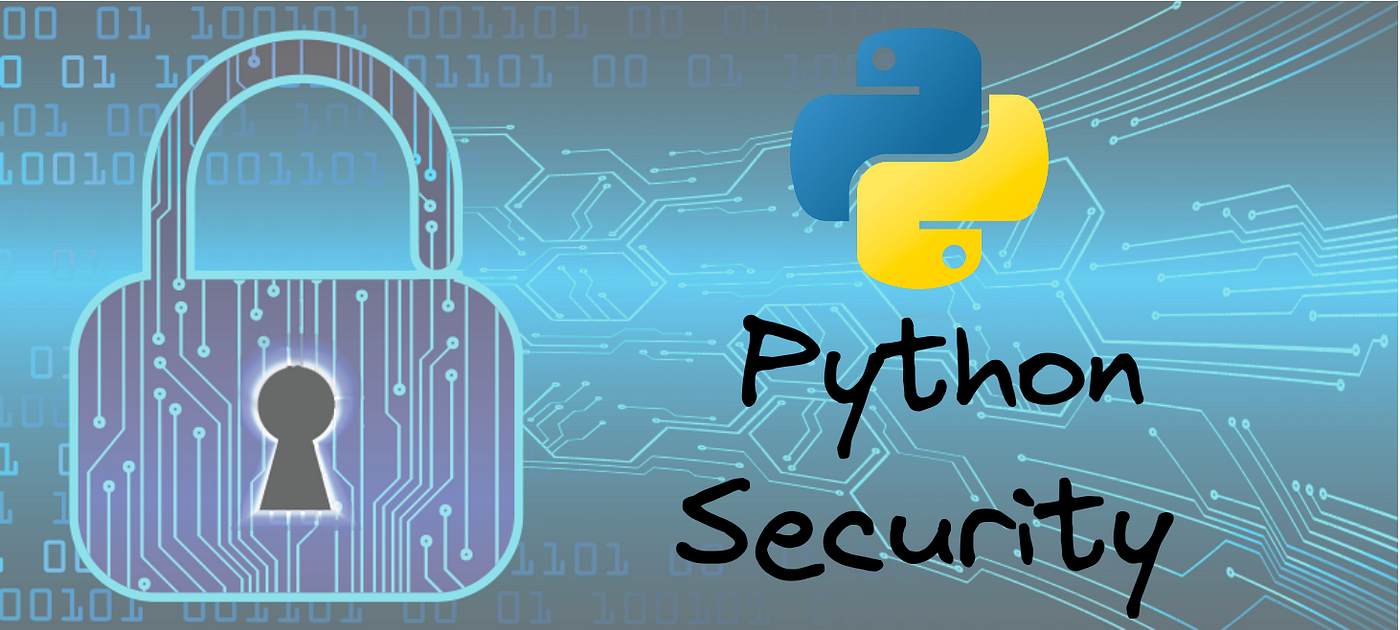
Python : SSL Version Scanner
Python package to find SSL/TLS version of a Host. You can modify the script for host, and get the SSL/TLS version number in order to find their vulnerabilities.
Follow this below link, to get your package ready :
After setting up, use this in your script to get the ssl/tls version number.
1) This is your __init__.py script
#! usr/bin/python
import socket
import nmap
def printSSL(h):
host = h
addrs = socket.gethostbyname(host)
nm = nmap.PortScanner()
nm.scan(hosts= addrs, arguments=’-n -sV –script ssl-enum-ciphers -Pn -p 443′)
ssl = nm[addrs][‘tcp’][443][‘script’][‘ssl-enum-ciphers’]
for item in ssl.split(“n”):
if “TLSv” in item:
version = item.strip()
print (“SSL/TLS Version of ” + str(host) + ” is %s” % version)
2) This is your setup.py script
from setuptools import setup
setup(name=’SSL_Scanner’,
version=’0.1′,
description=’Version Scanner’,
url=’http://maddog.com’,
author=’Hooman’,
author_email=’hooman@example.com’,
license=’CAT’,
packages=[‘SSL_Scanner’],
zip_safe=False)
3) Install twine to register the package
# pip install twine
4) Register your package
# python setup.py sdist
5) Upload your package to PYPI
# twine upload dist/*
6) pip install this package
# pip install SSL_Scanner
7) Use the package in your script
>>> import SSL_Scanner
>>> SSL_Scanner.printSSL(“yahoo.com”)
or
>>> SSL_Scanner.printSSL(“bingo.com”)
There you have it, above script(s) will give us the list of ssl/tls version number which can further be used to get the vulnerabilities of an host.
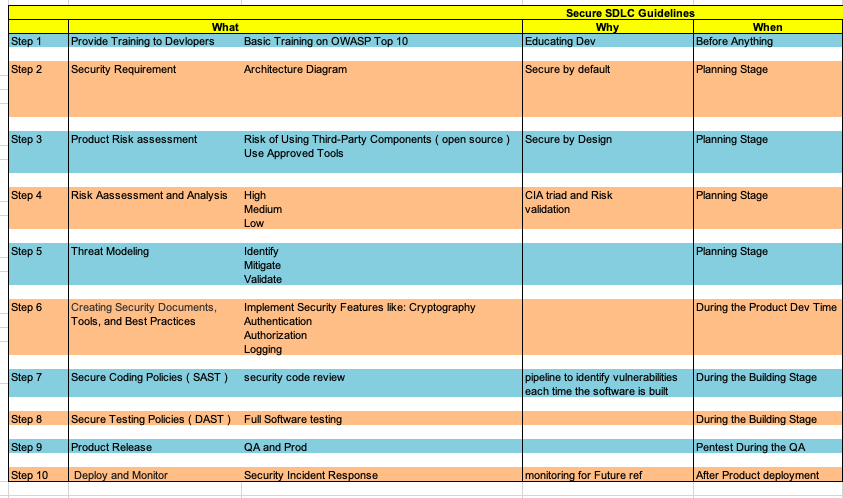
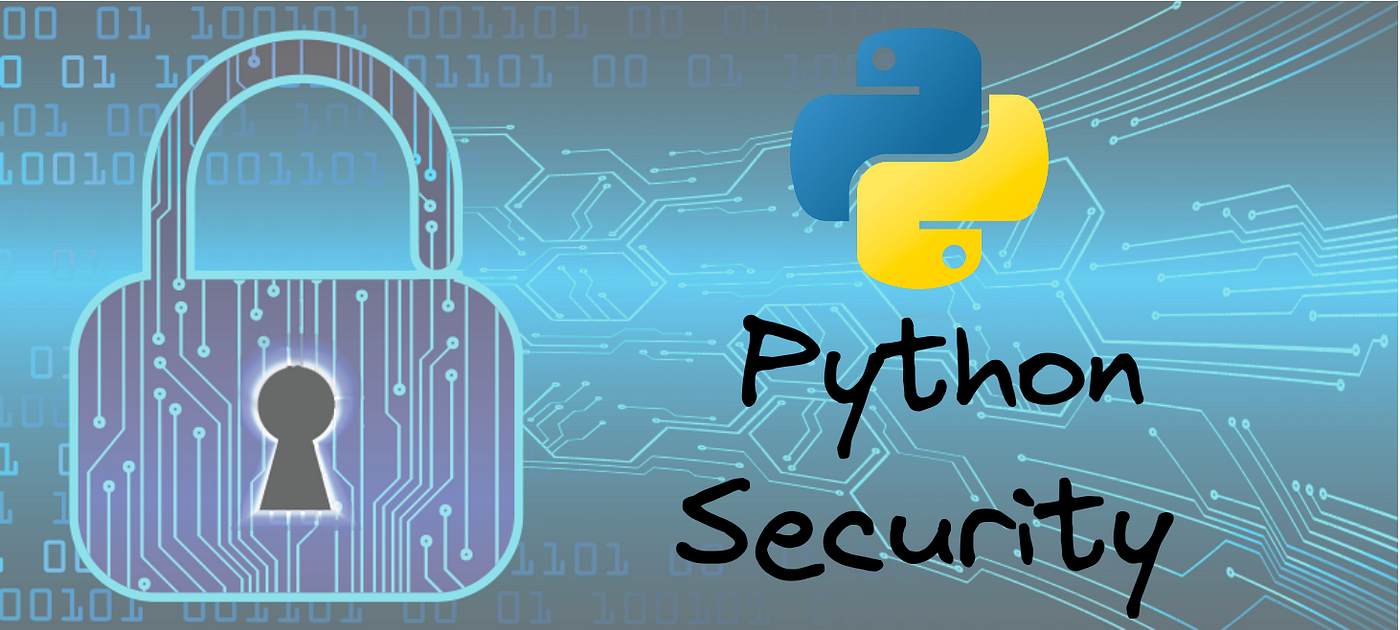